As a followup to the recently published post, "Creating a Geocoder Service Using Dreamfactory and the Google Maps Geocoding API", I thought it would be fun to continue expanding upon this theme and demonstrate how to create a geofence API using the Haversine equation and a DreamFactory PHP Scripted Service API.
A geofence is a virtual perimeter surrounding a location defined by a specific pair of latitudinal and longitudinal coordinates. For example, the map overlay found in the following screenshot simulates what a 500' radius geofence placed around the iconic Empire State Building would look like:
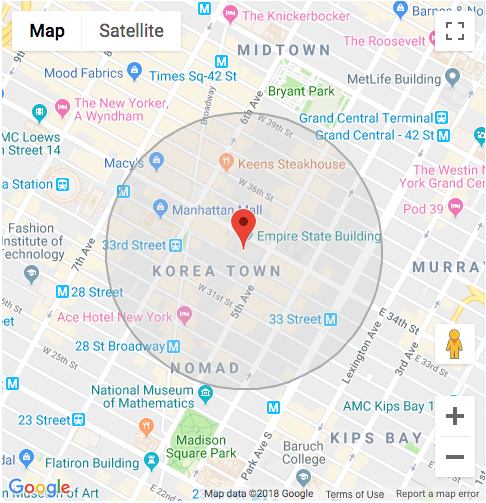
These days, geofences are commonly used for a wide variety of applications, including providing customers with localized coupons once they enter a store, wildlife management, and security.
In this article you'll learn how to insert the Haversine Equation into a scripted DreamFactory service. Once done you'll be able to issue a simple HTTP call to your DreamFactory instance, passing along two sets of coordinates in order to determine how far away a subject is from the target destination. Before doing so though, it's worth talking about the mathematical formula that makes this all possible, known as the Haversine formula.
Introducing the Haversine Formula
Wikipedia defines defines the Haversine formula as being used to "determine the great-circle distance between two points on a sphere given their longitudes and latitudes". The "great-circle distance" is the shortest distance between two points as measured on the surface of a sphere, which makes it a relatively accurate solution for measuring distance between two points on Earth.
This formula involves a fairly heavy dose of geometry, and so I'll leave it to the aforementioned Wikipedia page to walk you through the mathematical details. We'll instead focus on converting this formula into its' PHP equivalent, and making this functionality available via a secure DreamFactory service.
Did you know you can generate a full-featured, documented, and secure REST API in minutes using DreamFactory? Sign up for our free 14 day hosted trial to learn how! Our guided tour will show you how to create an API using an example MySQL database provided to you as part of the trial!
Access Your No-Code APIs Now
Creating the Geofence API
To create the Geofence API in DreamFactory, we'll begin by creating a new PHP Scripted Service. This is accomplished by logging into your DreamFactory instance, clicking on Services
, clicking Create
, and selecting Script
and finally PHP
(see below screenshot).
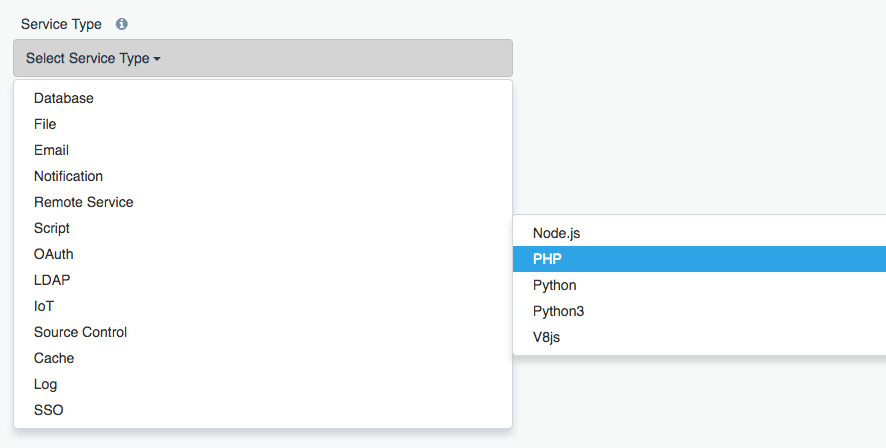
Assign a name, label, and description to the new service. I selected geofence
, Geofence API
, and Geofence API
, respectively. Next, click on the Config
tab, and in the text area add the following PHP code:
if ($event['request']['method'] == "GET") {
$lat1 = (float) $event['request']['parameters']['lat1'];
$lng1 = (float) $event['request']['parameters']['lng1'];
$lat2 = (float) $event['request']['parameters']['lat2'];
$lng2 = (float) $event['request']['parameters']['lng2'];
$radius = 3959;
$dLat = deg2rad($lat2 - $lat1);
$dLon = deg2rad($lng2 - $lng1);
$a = sin($dLat/2) * sin($dLat/2) + cos(deg2rad($lat1))
* cos(deg2rad($lat2)) * sin($dLon/2) * sin($dLon/2);
$c = 2 * asin(sqrt($a));
$d = $radius * $c;
return $d;
}
This is the Haversine formula implemented in PHP and wrapped around a GET
HTTP call listener. If you want to return the distance between points in kilometers instead of miles, replace 3959
with 6371
. Once saved, and after configuring an associated DreamFactory role and API key, you'll be able to call your API like so:
/api/v2/geofence?lat1=40.748667&lng1=-73.985667&lat2=40.753706&lng2=-73.982341
The 40.748667,-73.985667
coordinates identify the location of the Empire State Building, and the -73.985667,40.753706
coordinates identify the location of the New York Public Library (5th avenue branch). The service returns a distance of just 0.3892
miles; a short walk between these two famous landmarks!
Where to From Here?
Managing these sorts of API-based services inside DreamFactory is great for many reasons. Firstly, DreamFactory-managed services are reusable, meaning you won't have to bother with repeatedly coding the Haversine formula every time the need arises to insert this sort of functionality into an application. You can instead just quickly connect to the existing service!
Second, you can optionally incorporate various advanced DreamFactory features to suit application-specific needs:
- Use DreamFactory's limiting feature to restrict the number of hourly/daily/monthly calls a particular user can make, opening up the opportunity to monetize your data.
- Integrate the geofence API into another API's workflow, allowing you to return the distance between two points alongside other response data.
Conclusion
Would you like to see this example demonstrated in person? Contact our team to arrange a demo. Or download the OSS version of DreamFactory and experiment with this feature for yourself!