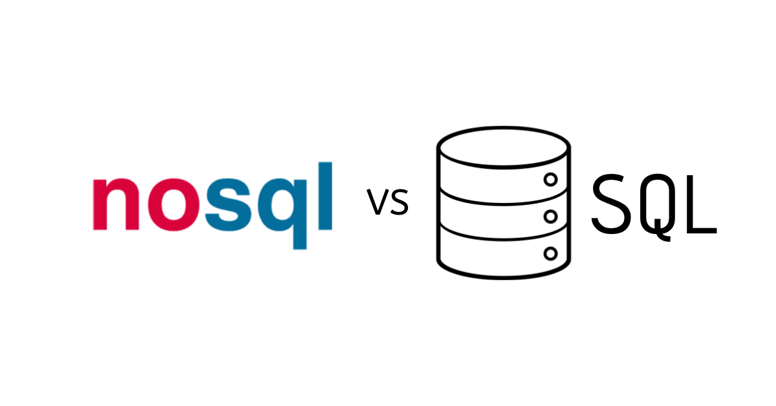
MySQL, API Management, Services, SQL, Uncategorized, DreamFactory, API Automation, NoSQL, Database, API
NoSQL vs SQL: Understand the Differences and Make the Best Choice
When it comes to choosing a database, one of the biggest decisions is picking a relational (SQL) or non-relational (NoSQL) data structure.
by Kevin McGahey • July 1, 2019
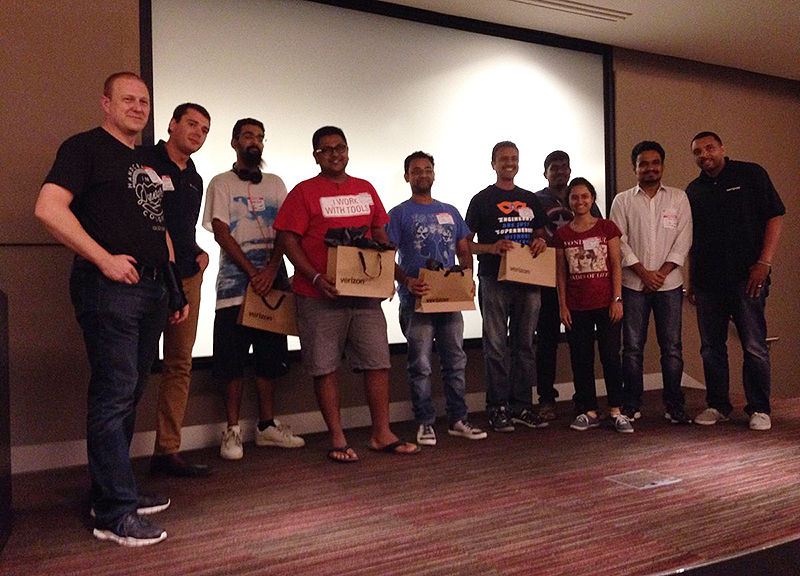
API Management, Services, DreamFactory, Meet Up, API Automation, NoSQL, Data Sources, API Design, SOAP, Database, Authentication, API
DreamFactory hackathon with Verizon Cloud and Mapquest
by Terence Bennett • July 6, 2018
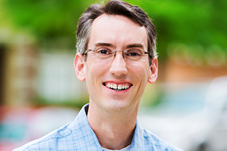
MySQL, API Management, Services, DynamoDB, SQL, security, Redshift, REST, AWS, DreamFactory, API Automation, Azure, NoSQL, MongoDB, Data Sources, API Design, SOAP, Database, Authentication, API, CouchDB
NoSQL, No Problem!
by Terence Bennett • July 6, 2018
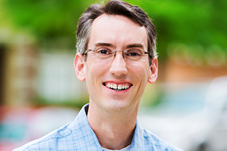
MySQL, SQL Server, API Management, Services, SQL, Redshift, REST, AWS, DreamFactory, API Automation, NoSQL, Data Sources, API Design, SOAP, Database, Microsoft SQL Server, Authentication, Microsoft Server, API
NoSQL, No Problem! - Operation Specifics
by Terence Bennett • July 6, 2018
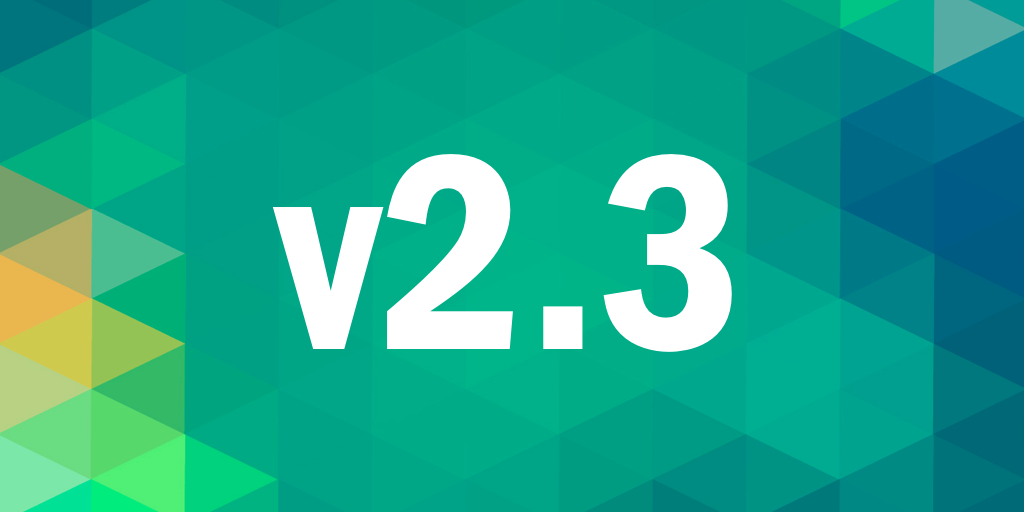
API Management, Services, SQL, REST, Swagger, DreamFactory, API Automation, NoSQL, Data Sources, API Design, Release Notes, Cassandra, Release Notes, SOAP, Database, Authentication, API Limits, API
DreamFactory 2.3 released, includes PHP 7, Cassandra, Redis
by Terence Bennett • July 6, 2018
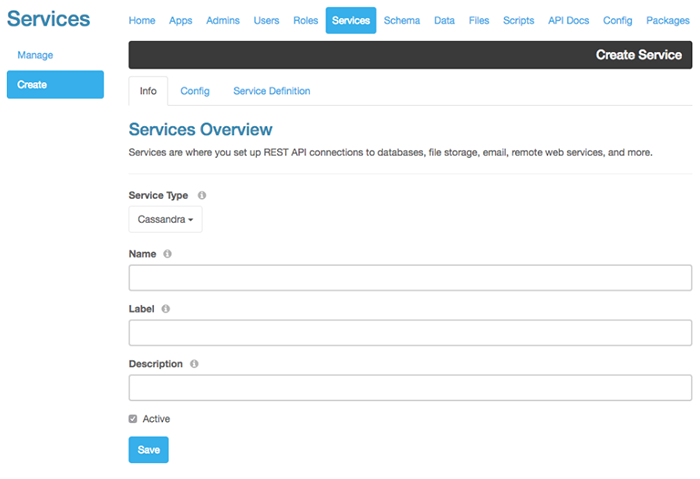
API Management, Services, REST, Swagger, DreamFactory, API Automation, NoSQL, Data Sources, API Design, Cassandra, SOAP, Database, Authentication, API Limits, API
Introducing the DreamFactory Cassandra Service
by Kevin McGahey • July 6, 2018
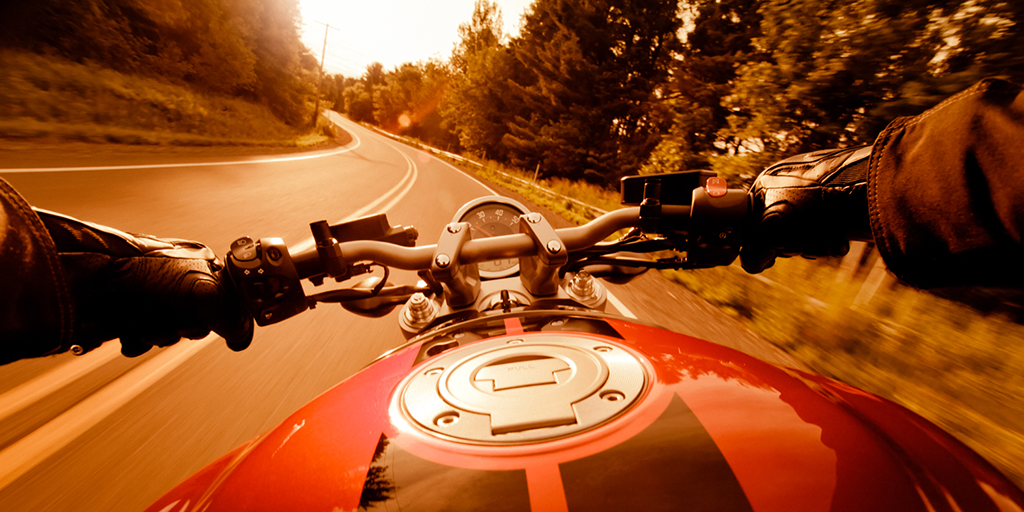
JSON, API Management, Services, SQL, REST, DreamFactory, API Automation, NoSQL, PHP, Data Sources, API Design, SOAP, Database, Authentication, API
PHP 7 doubles performance for DreamFactory
by Terence Bennett • July 6, 2018