Using OAuth with the New Portal Service (Part 2)
by Terence Bennett • July 6, 2018

Are we there yet?
This post picks up where part 1 left off. My original post was too long so I split it into two parts. In reviewing the second installment, I realized that the concepts were a bit vague and would be better conveyed through an actual application.
Thus, the Portal Sandbox was born! The full source code is available on GitHub. Go ahead and clone a copy so you can follow along. I'll wait...
If you landed here first, start at the beginning with our Part One in Using OAuth with the New Portal Service
First Installment Recap & Checklist
So in Part 1, I introduced the concept of the portal. As you will most certainly recall, the new Portal service provides centralized access to authenticated services and API on a per-user basis. To use the portal service, you must create Providers, or endpoints; and any associated keys for said provider(s).
Pssst...
Currently, the portal service only supports providers who accept OAuth 2 authentication. LDAP and OpenID are next to be integrated. In any case, if your provider authenticates via OAuth 2 you can access it via the portal service. With that said, for the purposes of this blog, Facebook's Graph API was chosen for the example provider.
When a user authenticates with a provider, the returned access credentials (an OAuth token in the case of Facebook) are encrypted and stored in the ProviderUser resource. These are accessible via the DSP's REST API using the /rest/system/provider
and /rest/system/provider_user
endpoints respectively. The following flow chart in figure 1 shows the process in broad strokes.

Confused? Don't be, it'll all become quite clear once we fire up the sandbox. With that said, let's recap the key concepts and make sure you've got all your ducks in a row.
Key Concepts from Part One:
- The new Portal service provides your DSP with access to authenticated services and APIs on a per-user basis.
- No providers are supplied with the DSP. You must create them yourself. And, if you want to play along at home, you must register as a Facebook developer (if you're not already), and create a new application for your DSP's portal. Go back and read part one for info on how this is done.
Related reading: DreamFactory & Facebook OAuth 2.0
Say What?!
Yes, sadly, no UI currently exists to manipulate providers or provider users. This will be available in a future update (at the time of this writing the current version is 1.3.3). So, you will need to create your providers through one of myriad methods including, but not limited to: curl, PHP script, in-browser REST clients, and/or IDE REST clients.
Here is a handy-dandy JSON template to use with your favorite client.
{
"api_name": "fbportal",
"provider_name": "Facebook",
"is_active": true,
"is_system": false,
"is_login_provider": false,
"config_text": {
"client_id": "PUT YOUR CLIENT ID HERE",
"client_secret": "PUT YOUR CLIENT SECRET HERE",
"scope": [
"user_about_me",
"email",
"user_birthday",
"user_groups"
]
}
}
Just be sure to replace the PUT YOUR CLIENT... HERE strings with actual values before you send the POST.
Breaking Change Notice
If you created this provider previously, please note the following change:
The provider.provider_name
value must be changed from Facebook Portal (from part one tutorial) to Facebook as shown in the template above. This field's value must match a provider template from the Oasys library. Currently, Oasys supports Facebook, GitHub, Salesforce, and a few others. If it is already set to Facebook, you're good to go.
- Each call to a portal service is relayed verbatim (less any DSP-specific parameters) to the provider.
A Real-World Example
Here is an actual portal call to retrieve the current user's profile from Facebook:
https://dsp-yours.cloud.dreamfactory.com/rest/portal/fbportal/<span class="str">me?app_name=admin</span>
The portal service translates this call into:
https://graph.facebook.com/<span class="str">me?access_token=<user's token="" inserted="" here="" for="" you=""></span>
The service makes this request, which is an HTTP GET, and returns the results:
{
"result": {
"id": "10929849342",
"name": "Rain Man",
"first_name": "Ray",
"last_name": "Monde",
"link": "https://www.facebook.com/rainman",
"username": "rainman",
"about": "I'm an excellent driver.",
"birthday": "06/06/1966",
"hometown": {
"id": "108659242498155",
"name": "Chicago, Illinois"
},
"location": {
"id": "107991659233606",
"name": "Atlanta, Georgia"
},
"bio": "I love Judge Wopner.",
"gender": "male",
"email": "rainman@example.com",
"timezone": -5,
"locale": "en_US",
"verified": true,
"updated_time": "2013-12-09T18:46:57+0000"
},
"code": 200,
"content_type": "application/json; charset=UTF-8"
}This data is a fictitious example if you hadn't figured that out.
That pretty much sums it up. In this installment, we are going to use the portal service to get some data.
Enter Sandbox (We're off to never never-land)
Besides finding free time to write, the major reason for the delay of this installment was software development. I wanted to provide a working application that used the portal service. In addition, I wanted to be able to build upon this example application for future blog posts. The end result is a small application called the Portal Sandbox.
The full source code is available on GitHub here: https://github.com/dreamfactorysoftware/portal-sandbox
If you've been developing with the DSP API, I'd wager that it was in Javascript/HTML with AJAX. This application, however, is a bit of a hybrid. It piggy-backs on the DSP's server core to leverage direct database access via the ResourceStore object from our PHP Platform SDK.
The ResourceStore and Custom Resources
In my next blog I will be writing about the ResourceStore and how to extend it to add business rules to your resources. The Portal Sandbox will be utilized to add some logic around Salesforce data manipulation.
If you want to play along at home, you need to clone the Portal Sandbox app repo into your DSP's /web
directory. This application will not work if installed into the default "application" space. The reason being is that no server-side code is currently executed if stored there. Only straight-up HTML is rendered and thus, only client-side code is available. /web
is your DSP's document root directory. Once you've cloned the app, add it to your DSP via the Admin console as a remote URL application and set the URL to /portal-sandbox/index.html
.
Please note that this application must be run on a stand-alone (i.e. platform package or BitNami stack) DSP. Hosted DSPs cannot use this application. If you're a new developer or at a loss here, check out Vagrant for easy development server management.
Full installation instructions are as follows:
- Clone and Install
- Change to your DSP's
/web
directory. - Issue the clone command:
git clone https://github.com/dreamfactorysoftware/portal-sandbox.git .
- Change into the new
portal-sandbox
directory. - Issue the following command:
php ../../composer.phar install
- Change to your DSP's
- Create the Application
- Log into your DSP as an administrator
- Click on the "Apps" section and add the Portal Sandbox app and save
- Go back into the newly created app and change where the app lives to "I will supply an URL"
- Change the URL to
/portal-sandbox/index.php
and save - Add a provider to your DSP if you haven't already
Now when you click on your DSP's application list, you will see the sandbox app in the list.

Opening the sandbox shows the main screen of the sandbox app as shown in figure 3.

Who Are You?
If you've created a provider you should see it selected in the drop-down. Next to it is a link to authorize. Click this link to begin the Facebook authentication process. This will redirect you to the generic Facebook login page as shown in figure 4. Enter your credentials and click Log In to authenticate and continue.
The next page will ask you to authorize the application to have access to your account. Change anything you'd like and press the Allow button.

The page will redirect back to your DSP and automatically load the Portal Sandbox application. What you should see is shown in figure 5.

Portals and Beyond...
This short series has provided only a glimpse of what the new portal service can do. Access to remote APIs with secure credential storage handled for your app automatically? Uhm, yeah! And if you are anything like me, I'm sure your mind is awash with the possibilities this powerful tool can give to you and your applications.
Good luck finding your way to the other side!
Start at the beginning with our guide to using DreamFactory 2.0 with OAuth Services
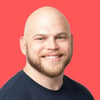
Terence Bennett, CEO of DreamFactory, has a wealth of experience in government IT systems and Google Cloud. His impressive background includes being a former U.S. Navy Intelligence Officer and a former member of Google's Red Team. Prior to becoming CEO, he served as COO at DreamFactory Software.
Me, Myself, and My Friends
Now you have access to Facebook's Graph API via the portal service for the authorized user. So, let's exercise the Graph API by pulling the friends list of this user.
The second section of the sandbox application, Call Settings, allow you to experiment with the provider API. The sandbox has no knowledge of the API specifics so it is up to you to provide the proper endpoints and parameters (other than the OAuth parameters that is). By default, the app sets the endpoint to the identity or profile URL of the currently selected provider. For Facebook, this endpoint is
/me
. Go ahead and click the Send Request button to make the call. Figure 6 shows what my account returns./me
Now, let's see my friends. Change the endpoint from
/me
to/me/friends
, then click Send Results. As you can see in figure 7, I have no friends. :( Hopefully, you'll have some returned./me/friends
As an extra-added bonus, the sandbox app displays the total elapsed time for the call. This is the full round-trip time. It's more eye candy than anything else, but definitely shows that the portal service doesn't add too much latency to your API calls.