Error Handling in SOAP to REST: Best Practices
by Kevin McGahey • May 19, 2025Did you know that 20% of API calls result in errors during SOAP to REST migrations? Poor error handling can lead to data loss, system failures, and degraded performance. Here's how to manage errors effectively during migration:
- SOAP vs. REST Differences: SOAP uses standardized faults (XML), while REST relies on HTTP status codes (JSON).
- Key Challenges: Mapping SOAP faults to REST codes, XML-to-JSON conversion, and managing state in stateless REST.
- Solutions: Map SOAP faults to REST codes (e.g., 401 for authentication errors), use structured JSON for error responses, and implement robust logging and testing.
Quick Comparison
Feature |
SOAP |
REST |
---|---|---|
Error Format |
Standardized SOAP Faults |
HTTP Status Codes |
Message Structure |
XML-based, verbose |
Flexible (JSON, XML) |
State Management |
Stateful operations |
Stateless by default |
Start by aligning error codes, ensuring detailed logging, and testing thoroughly to avoid disruptions. A single unhandled error can cause significant downtime, as seen in past incidents.
BEYOND Status Codes! Better REST HTTP API Error Responses
Error Handling Methods in SOAP and REST
Grasping the nuances of error handling in SOAP and REST is essential when navigating API migrations. Let’s break down how each protocol approaches errors and how to handle them effectively.
SOAP Fault Components and Examples
SOAP follows a structured approach to error reporting, relying on XML-formatted fault messages. Each SOAP message is limited to one fault block within its body element [1]. The fault structure differs slightly between SOAP 1.1 and 1.2, with each version using specific elements for error descriptions.
SOAP 1.1 Element |
SOAP 1.2 Element |
Purpose |
---|---|---|
|
|
Specifies the type of error (Required) |
|
|
Provides a human-readable error message (Required) |
|
|
Identifies the source of the error (Optional) |
|
|
Contains application-specific error details (Optional) |
Here’s an example of a SOAP fault message, typically seen during a failed credit card validation process:
<SOAP-ENV:Fault>
<faultcode>SOAP-ENV:Client</faultcode>
<faultstring>Failed to locate method (ValidateCreditCard) in class (examplesCreditCard) at /usr/local/ActivePerl-5.6/lib/site_perl/5.6.0/SOAP/Lite.pm line 1555.</faultstring>
</SOAP-ENV:Fault>
SOAP’s detailed fault structure ensures clarity, but REST takes a different, more streamlined approach.
REST Error Response Guidelines
REST APIs rely on HTTP status codes paired with structured responses to communicate errors. These status codes are grouped into categories that quickly indicate the error’s nature:
Status Range |
Purpose |
Common Use Cases |
---|---|---|
400-499 |
Client Errors |
Issues like authentication failures or invalid input |
500-599 |
Server Errors |
Problems such as database failures or unavailable services |
A well-structured REST error response might look like this:
{
"status": "error",
"statusCode": 404,
"error": {
"code": "RESOURCE_NOT_FOUND",
"message": "The requested resource was not found.",
"details": "The user with the ID '12345' does not exist in our records.",
"timestamp": "2023-12-08T12:30:45Z"
}
}
REST’s approach is clean and concise, making it easier for developers to understand and debug issues.
Converting SOAP Faults to REST Status Codes
When moving from SOAP to REST, it’s crucial to map SOAP faults to REST’s HTTP status codes while preserving error details for traceability. Here’s how to approach this process:
- Map fault codes: Align SOAP fault codes with the most appropriate HTTP status codes.
- Transform error details: Convert SOAP’s
detail
element into a structured JSON format. - Maintain traceability: Ensure error identifiers and details remain consistent across the migration.
- Standardize logging: Implement uniform error logging to simplify debugging.
For added consistency, REST error responses should adhere to RFC 9457 (Problem Details) [3], which recommends including the following fields:
- type: A URI identifying the error category.
- title: A brief, human-readable summary of the error.
- status: The corresponding HTTP status code.
- detail: A more in-depth description of the error.
- instance: A unique identifier for this specific error occurrence.
Error Handling Problems in API Migration
Moving from SOAP to REST APIs brings its own set of technical hurdles, especially when it comes to error handling. Let’s break down the key challenges and their implications.
Protocol Conversion Challenges
A significant issue during migration is the disparity in how SOAP and REST handle faults. For instance, 75% of SOAP users encounter interoperability issues due to improper fault handling [4]. Here’s a closer look at the main protocol-related problems:
Challenge |
Impact |
Solution Approach |
---|---|---|
Endpoint Mismatches |
Causes 60% of interoperability failures |
Enforce strict endpoint validation and mapping |
Status Code Confusion |
Leads to 45% of communication problems |
Develop detailed status code conversion matrices |
WSDL Specification Conflicts |
Accounts for 35% of integration issues |
Implement clear WSDL version control and validation |
These challenges often arise because REST lacks the rigid structure of SOAP, making it more prone to misalignments during conversion.
Data Format Errors
Transitioning from XML to JSON is another common stumbling block, as 74% of integration failures stem from message structure discrepancies [4]. Two primary areas where data format errors occur are:
- XML Schema Validation:
Over half (55%) of data-related issues are linked to invalid XML structures [4]. This becomes especially problematic when dealing with complex data types that don’t have straightforward JSON equivalents. - Namespace Handling:
About 40% of interoperability challenges involve namespace conflicts [4]. SOAP’s hierarchical namespace structure doesn’t translate seamlessly into REST’s simpler format, creating room for errors.
For example, in March 2025, a financial services company faced significant integration failures when its intricate XML schemas clashed with a third-party insurance provider’s simpler structure. This mismatch disrupted over 20% of their transactions [4].
State Management in REST
SOAP and REST differ fundamentally in how they manage state. While SOAP relies on a stateful approach, REST operates in a stateless manner, which introduces unique challenges:
State Management Aspect |
SOAP Approach |
REST Solution |
---|---|---|
Session Data |
Stored on the server |
Managed on the client |
Request Context |
Maintained between calls |
Encapsulated within each request |
Processing Speed |
Faster with session data |
Slower due to additional validation |
Scalability |
Limited by state storage |
Improved by stateless architecture |
To bridge this gap, organizations need to:
- Use token-based authentication for session management.
- Leverage client-side storage mechanisms effectively.
- Design self-contained requests that include all necessary context.
It’s worth noting that 30% of integration issues arise from misinterpretations of fault messages [4]. Creating a robust plan for managing state during migration is crucial to avoid these pitfalls and maintain reliable error handling. The next section will explore precise strategies for overcoming these challenges.
Error Handling Guidelines for API Migration
Tackling the protocol and data challenges in API migration means setting up clear error-handling practices.
Creating Error Code Mapping Rules
Start by aligning SOAP faults with appropriate REST status codes. Here’s a sample mapping:
SOAP Fault |
REST Status Code |
Usage Scenario |
---|---|---|
Client.Authentication |
401 Unauthorized |
Invalid or missing credentials |
Client.ResourceNotFound |
404 Not Found |
Requested resource doesn't exist |
Server.ValidationError |
400 Bad Request |
Invalid input parameters |
Server.DatabaseError |
500 Internal Server Error |
Database connection issues |
Server.Timeout |
504 Gateway Timeout |
Service request timeout |
For JSON error messages, maintain a structured format like this:
{
"status": "error",
"statusCode": 404,
"error": {
"code": "RESOURCE_NOT_FOUND",
"message": "The requested resource was not found.",
"details": "The user with the ID '12345' does not exist in our records.",
"timestamp": "2025-05-19T14:30:45Z",
"path": "/api/v1/users/12345"
}
}
Error Logging and Tracking
When logging errors, capture critical details such as:
- The error type and severity
- Request and response data
- Timestamps and transaction IDs
- User context and affected resources
- Stack traces for server-side issues
Research shows that companies with robust logging practices see a 40% drop in incident resolution times [5]. Use a consistent logging format like this:
{
"level": "ERROR",
"timestamp": "2025-05-19T14:30:45Z",
"service": "user-authentication",
"event": "login_failure",
"error_code": "AUTH_001",
"message": "Invalid credentials provided",
"request_id": "abc123xyz",
"user_id": "user_12345"
}
Once the logging system is in place, test its reliability through thorough evaluations.
Error Handling Tests
Conduct testing in three main areas to ensure robust error handling:
Validation Testing
- Check for invalid authentication tokens.
- Test malformed request payloads and resource conflicts.
- Simulate network timeouts.
Integration Testing
- Verify consistent error message formatting.
- Ensure proper status code mapping.
- Test error logging and response time monitoring.
Load Testing
- Measure error response times under stress.
- Assess system resource usage and log storage capacity.
- Monitor the accuracy of error tracking.
Always ensure error responses are secure and do not expose sensitive internal details. Tools like DreamFactory can help streamline SOAP to REST migrations while improving error reliability.
Conclusion
Main Points Review
Handling errors effectively during a SOAP to REST migration is essential for maintaining system reliability, stability, and reducing debugging time [6]. Here’s a quick recap of the key strategies:
Focus Area |
Best Practice |
Impact |
---|---|---|
Error Mapping |
Consistent SOAP to REST conversion |
Uniform error reporting across systems |
Response Format |
Structured JSON with detailed info |
Clear communication for API consumers |
Monitoring |
Comprehensive logging and tracking |
Faster and more efficient error resolution |
Security |
Protect internal system details |
Reduced risk of security vulnerabilities |
"Error handling is a crucial part of working with APIs" [2] - Gbadebo Bello, Developer Relations Engineer at Postman
These insights lay the foundation for implementing robust error management practices during migration.
Implementation Guide
Here’s how to put these principles into action:
Define Error Standards
Design consistent error response formats, map SOAP faults to appropriate HTTP status codes, and use retry mechanisms like exponential backoff to handle transient issues effectively.
Establish Monitoring Systems
Set up detailed logging to track errors, monitor response times, and evaluate API performance to quickly identify and resolve issues.
A real-world example underlines the importance of these practices: Netflix reported that a single unhandled error in their recommendation API caused a 45-minute outage, disrupting service for millions in 2022 [7].
To simplify the process, tools like DreamFactory offer automated API generation with built-in error handling and standardized response formats. These solutions help ensure consistent error management, making your migration from SOAP to REST smoother and more secure.
FAQs
What challenges arise when mapping SOAP faults to REST status codes during API migration?
Mapping SOAP faults to REST status codes can be a challenging part of API migration because of the contrasting ways these two systems handle errors. SOAP uses well-defined fault codes that offer detailed descriptions of issues, while REST leans on HTTP status codes, which are typically more general. This difference can make it hard to translate SOAP faults into REST responses without losing important details.
To bridge this gap, developers often resort to creating custom JSON error responses that mirror the level of detail provided by SOAP. While this approach can help preserve clarity, it also adds complexity and increases the chance of miscommunication between the client and server. Maintaining clear, consistent, and informative error handling throughout the migration process is essential to ensure the system remains reliable and efficient.
What are the best practices for effective error logging when migrating from SOAP to REST?
To keep error logging on point during a SOAP to REST migration, it’s crucial to adopt structured logging. This means including key details like timestamps, error types, and relevant contextual data. Such an approach makes tracking and diagnosing issues much more straightforward.
Another smart move is to use a centralized logging solution. This pulls logs from all your services into one place, making real-time monitoring a breeze and helping you quickly spot recurring problems. Also, be sure to define clear logging levels - like DEBUG, INFO, and ERROR - so you can filter logs based on their importance. That way, critical issues won’t get buried under less urgent details.
These strategies can make the migration process smoother by improving reliability and simplifying troubleshooting.
How can you effectively manage state in a stateless REST environment after migrating from a stateful SOAP architecture?
When transitioning from a stateful SOAP architecture to a stateless REST environment, managing state effectively becomes a key challenge. One widely used method is token-based authentication, such as JSON Web Tokens (JWT). With this approach, the client includes session details as part of each request. This keeps the server stateless while still preserving session continuity.
Another option is leveraging external state management solutions like caching systems or databases. These tools allow you to store session data externally, enabling the server to fetch the required state information when needed, all while adhering to REST's stateless design principles. By combining these techniques, you can ensure your REST APIs remain both efficient and dependable after migration.
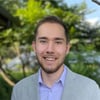
Kevin McGahey is an accomplished solutions engineer and product lead with expertise in API generation, microservices, and legacy system modernization, as demonstrated by his successful track record of facilitating the modernization of legacy databases for numerous public sector organizations.