CORS Issues in API Development: Simplifying Cross Origin Request
by Kevin McGahey • October 24, 2024Nowadays, building robust and secure RESTful APIs is a fundamental task. However, developers often stumble upon a common challenge: Cross-Origin Resource Sharing (CORS) issues. These issues can hinder the integration of APIs from across different domains, leading to frustration and delays in development timelines.
In this article, we’ll be discussing the intricacies of CORS, and figuring out why these security features are essential. Then we’ll be exploring how DreamFactory effectively eliminates CORS-related hurdles.
- CORS (Cross-Origin Resource Sharing) is a security feature in web browsers that controls interactions between web applications and resources from different domains to prevent unauthorized access.
- The Same-Origin Policy (SOP) restricts how documents and scripts interact across domains, but CORS allows controlled cross-origin requests by defining rules on who can access resources.
- Common CORS issues include missing or misconfigured headers, preflight request failures, and difficulties handling credentials or cookies in cross-origin requests.
- CORS security features exist to protect user data from cross-site scripting attacks and unauthorized access, ensuring secure interactions between web applications and external APIs.
- DreamFactory simplifies CORS management by automating CORS configurations, managing preflight requests, and providing security-focused default settings to reduce errors and streamline API development.
Understanding the CORS Conundrum
What is CORS?
Cross-Origin Resource Sharing (CORS) is a security mechanism enforced by web browsers to control how web applications interact with resources hosted on different origins (domains, protocols, or ports). Essentially, it governs whether a web page can make requests to a domain other than the one that served the web page.
The Same-Origin Policy
At the heart of CORS lies the Same-Origin Policy (SOP), a critical security concept implemented in browsers to prevent malicious scripts on one page from obtaining access to sensitive data on another page through the browser's Document Object Model (DOM). The SOP restricts how a document or script loaded from one origin can interact with resources from another origin.
The CORS Mechanism
CORS extends the SOP by allowing servers to specify who can access their resources and how. It uses a set of HTTP headers to determine whether a cross-origin request should be allowed. When a browser makes a cross-origin request, it includes an Origin header, and the server responds with Access-Control-Allow-Origin if it permits the request.
Common CORS Issues Faced by Developers
1. Blocked Requests Due to Missing Headers
One of the most frequent issues is the browser blocking requests because the server's response lacks the necessary CORS headers. Without the Access-Control-Allow-Origin header, the browser assumes the request is not permitted.
Example Error Message:
csharp
Access to XMLHttpRequest at 'https://api.example.com/data' from origin 'https://app.exampleclient.com' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
2. Incorrect Header Configuration
Sometimes, servers misconfigure the CORS headers, such as specifying the wrong domain in Access-Control-Allow-Origin or improperly setting Access-Control-Allow-Methods and Access-Control-Allow-Headers.
4. Credentials and Cookies Issues
When requests involve authentication (credentials or cookies), the server must explicitly allow credentials by setting Access-Control-Allow-Credentials: true, and the Access-Control-Allow-Origin header cannot be a wildcard (*).
The Preflight Request Issue (and Solution)
One of the most common issues developers face when working with cross-origin requests is the failure of preflight requests. This occurs when a browser, prior to sending a non-simple request (like POST, PUT, or DELETE), sends an OPTIONS request to the server to check if it’s allowed to make the actual request. If the server doesn’t respond with the correct CORS headers, the browser blocks the request, leading to errors like “No 'Access-Control-Allow-Origin' header is present on the requested resource.” This issue is frequently discussed in developer forums, such as this Stack Overflow post, where developers struggle with configuring the appropriate headers.
Manually handling these preflight requests can be a frustrating process. It means you need to pay careful attention to ensure that every response includes the right CORS headers (Access-Control-Allow-Origin, Access-Control-Allow-Methods, etc.) and that the server correctly manages both preflight and subsequent requests. Small misconfigurations can lead to development delays and blocked API calls, forcing developers to spend significant time troubleshooting.
DreamFactory eliminates this headache by automating the entire CORS handling process. Out of the box, DreamFactory:
- Automatically responds to preflight OPTIONS requests with the necessary CORS headers, ensuring that all cross-origin requests are allowed if they match the configured rules.
- Applies the appropriate Access-Control-Allow-Origin, Access-Control-Allow-Methods, and Access-Control-Allow-Headers consistently across all API responses.
- Allows developers to configure and customize these settings in its user-friendly administrative interface, without requiring them to manually handle headers for every request.
Since DreamFactory automates these configurations,it not only saves developers time but also reduces the potential for errors. Developers no longer have to repeatedly test and tweak their server-side CORS settings to ensure that their APIs are accessible across different domains. Instead, DreamFactory ensures that cross-origin requests—including those that require preflight checks—are handled smootly, allowing developers to focus on core application functionality.
Why Do These Security Features Exist?
Protecting User Data and Preventing Malicious Attacks
The primary purpose of CORS and the Same-Origin Policy is to protect users from Cross-Site Request Forgery (CSRF) and other malicious attacks that could compromise sensitive data. By restricting how resources are shared between origins, browsers prevent unauthorized scripts from accessing or manipulating data on another domain.
Ensuring Secure Web Interactions
CORS ensures that only trusted origins can access resources, which is crucial for maintaining the integrity and security of web applications. It provides a controlled way to relax the SOP, letting servers specify who can interact with their resources.
The Developer's Dilemma
Balancing Security and Functionality
Developers need to create applications that interact with APIs, often hosted on different domains. However, navigating the complexities of CORS configurations can be a time-consuming and error-prone process, especially when dealing with multiple APIs or services.
The Overhead of Manual Configuration
Manually setting up CORS headers requires careful attention to detail to avoid security vulnerabilities or functionality issues. Mistakes in configuration can lead to blocked requests or, worse, expose the application to security risks.
How DreamFactory Eliminates CORS Issues
Introduction to DreamFactory
DreamFactory is a powerful open-source REST API backend that automatically generates fully documented REST APIs for your data sources. It supports a wide array of databases and services, simplifying the process of API creation and management.
Automatic CORS Handling
One of DreamFactory's standout features is its ability to automatically handle CORS configurations out of the box. Here's how it addresses the common CORS challenges:
1. Default CORS Settings
DreamFactory comes with sensible default CORS settings that allow cross-origin requests from any domain. It sets the Access-Control-Allow-Origin header to *, enabling any origin to access the API.
Example Default Headers:
mathematica
Access-Control-Allow-Origin: *
Access-Control-Allow-Methods: GET, POST, PUT, PATCH, DELETE, OPTIONS
Access-Control-Allow-Headers: Content-Type, X-Requested-With
2. Customizable CORS Configurations
Developers can fine-tune the CORS settings through DreamFactory's administrative interface or configuration files. This flexibility allows for specifying allowed origins, methods, headers, and credentials according to the application's security requirements.
Configuring Allowed Origins:
In the DreamFactory admin panel, you can navigate to the CORS settings and specify which origins are permitted:
arduino
Allowed Origins: https://app.exampleclient.com
3. Support for Credentials
If your application requires sending credentials or cookies, DreamFactory allows you to enable Access-Control-Allow-Credentials with a simple configuration change.
Enabling Credentials:
yaml
Access-Control-Allow-Credentials: true
Benefits of Using DreamFactory for CORS Management
Saves Time and Reduces Errors
By automating CORS configurations, DreamFactory saves developers the time and effort required to manually set up and troubleshoot these settings. It reduces the likelihood of errors that can lead to security vulnerabilities or functional issues.
Enhances Security
DreamFactory's default configurations are designed with security best practices in mind. By providing a centralized place to manage CORS settings, it helps maintain a secure environment without sacrificing functionality.
Simplifies API Development
With CORS issues out of the way, developers can focus on the core aspects of API development, such as implementing business logic, optimizing performance, and enhancing user experience.
Practical Example: From Problem to Solution
Scenario: Building a Web Application with a Custom API
Suppose you're developing a web application hosted at https://app.exampleclient.com, and you've custom-built a REST API hosted at https://api.example.com. When the web application tries to make AJAX requests to the API, you encounter CORS errors.
The CORS Issue
The browser blocks the requests because https://api.example.com is a different origin from https://app.exampleclient.com, and the server hasn't configured the necessary CORS headers.
Error Message:
c#
Access to fetch at 'https://api.example.com/data' from origin 'https://app.exampleclient.com' has been blocked by CORS policy.
Attempted Solutions
You try to manually set the Access-Control-Allow-Origin header on your API responses, but you run into issues with preflight requests and handling credentials. The complexity increases as you need to support multiple HTTP methods and custom headers.
Introducing DreamFactory
DreamFactory allows you to completely eliminate these CORS issues effortlessly.
Step 1: Connect Your Data Source
In DreamFactory, connect your existing database or data source. DreamFactory will automatically generate RESTful endpoints for your data.
Step 2: Configure CORS Settings
Navigate to the Config section and adjust the CORS settings to match your application's needs.
- Allowed Origins: https://app.exampleclient.com
- Allowed Methods: GET, POST, PUT, PATCH, DELETE, OPTIONS
- Allowed Headers: Content-Type, X-Requested-With, Authorization
- Allow Credentials: true
Step 3: Update Your Application
Update your web application to point to the DreamFactory-generated API endpoints.
The Outcome
With DreamFactory handling the CORS configurations, your web application can successfully make cross-origin requests to the API without encountering CORS errors. Preflight requests are managed automatically, and credentials are handled securely.
Additional Features Beneficial to Developers
API Key Management
DreamFactory provides robust API key management, allowing you to control access to your APIs. You can create multiple API keys with different permissions, enhancing security and flexibility.
Role-Based Access Control
With role-based access control (RBAC), you can define roles and permissions for different users or applications, w that only authorized entities can access specific resources or perform certain actions.
Logging and Monitoring
DreamFactory offers detailed logging and monitoring capabilities, enabling you to track API usage, identify potential issues, and optimize performance.
Support for Multiple Data Sources
Whether you're working with SQL databases, NoSQL databases, file storage, or external web services, DreamFactory can integrate them, providing a unified API layer.
Conclusion
CORS issues are a common obstacle in web and API development, often causing significant delays and frustrations. However, manually configuring and managing CORS settings can be tedious.
DreamFactory is a powerful solution that automates CORS handling, letting developers to bypass these issues. DreamFactory takes care of CORS configurations, offers flexible settings, and includes strong security features, so developers can concentrate on building great applications without worrying about cross-origin issues.
FAQ: CORS and DreamFactory
1. What is CORS and why is it important?
CORS (Cross-Origin Resource Sharing) is a security mechanism enforced by web browsers that controls how web applications interact with resources hosted on different domains. It is important because it prevents unauthorized scripts from accessing or manipulating data on another domain, thereby protecting user data from cross-site scripting attacks and other malicious activities.
2. What is the Same-Origin Policy (SOP) in relation to CORS?
The Same-Origin Policy (SOP) is a security concept that restricts web pages from making requests to a different domain than the one that served the page. CORS extends SOP by allowing servers to specify who can access their resources and under what conditions, relaxing the SOP in a controlled way.
3. What are some common CORS issues faced by developers?
- Blocked Requests Due to Missing Headers: Browsers block requests if the server's response lacks necessary CORS headers, such as Access-Control-Allow-Origin.
- Incorrect Header Configuration: Misconfiguration of CORS headers, such as wrong domains in Access-Control-Allow-Origin or incorrect Access-Control-Allow-Methods.
- Preflight Request Failures: For non-simple requests, browsers send a preflight OPTIONS request. If the server doesn't handle this correctly, the actual request fails.
- Credentials and Cookies Issues: When using authentication (credentials or cookies), the server must set Access-Control-Allow-Credentials: true and cannot use a wildcard * for Access-Control-Allow-Origin.
4. Why do CORS security features exist?
CORS security features exist to protect user data from unauthorized access and to prevent malicious attacks, such as Cross-Site Request Forgery (CSRF). They ensure secure interactions between web applications and external APIs, allowing servers to control which origins can access their resources.
5. What challenges do developers face when handling CORS?
Developers often struggle to balance security with functionality. Configuring CORS headers manually can be complex and time-consuming, leading to potential errors, blocked requests, or even security vulnerabilities. This complexity is especially true when dealing with multiple APIs or services across different domains.
6. How does DreamFactory simplify CORS handling?
DreamFactory automates CORS configurations out of the box. It provides default settings that allow cross-origin requests from any domain and can be customized through its administrative interface. DreamFactory automatically manages preflight OPTIONS requests, preventing common failures, and supports credentials with a simple configuration change.
7. What are the benefits of using DreamFactory for CORS management?
- Saves Time and Reduces Errors: Automates CORS configurations, reducing the need for manual setup and troubleshooting.
- Improves Security: Provides sensible default settings based on security best practices, allowing fine-tuned control over allowed origins, methods, and headers.
- Simplifies API Development: Eliminates CORS-related issues, enabling developers to focus on business logic, performance, and user experience.
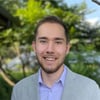
Kevin McGahey is an accomplished solutions engineer and product lead with expertise in API generation, microservices, and legacy system modernization, as demonstrated by his successful track record of facilitating the modernization of legacy databases for numerous public sector organizations.