API Caching Strategies, Challenges, and Examples
by Spencer Nguyen • December 10, 2024Imagine a user waiting several seconds for an app to load due to slow API responses. Frustration builds, user retention plummets, and scaling becomes a nightmare. API performance is a critical determinant of user experience and system reliability. Fast APIs ensure seamless interactions and support scalability. Among the strategies to enhance API speed, caching stands out as a powerful, cost-efficient approach.
What is Data Caching?
Data caching is the process of temporarily storing frequently accessed data in a faster storage layer to reduce the need for repetitive and resource-intensive operations. In the context of APIs, caching ensures quick retrieval of data, bypassing the need for database queries or complex computations on every request.
Types of Caches
- In-Memory Caches:
- Examples: Redis, Memcached.
- Store data in RAM, providing near-instantaneous access.
- Ideal for frequently accessed data requiring low latency.
- Distributed Caches:
- Spread across multiple nodes for scalability.
- Useful in environments where caching needs to support high availability and large-scale systems.
- Client-Side vs. Server-Side Caching:
- Client-Side: Stores data on the client (e.g., browser cache).
- Server-Side: Maintains cached data at the backend for centralized management.
Benefits of Caching for API Performance
- Improved Scalability: Caching reduces the load on the database by serving repeated queries from the cache. This enables the system to handle more concurrent users without scaling database infrastructure.
- Reduced Latency: Cached data retrieval is significantly faster than querying a database, leading to quicker API responses.
- Decreased Database Load: By minimizing direct queries, caching enhances database performance and reduces the risk of resource contention.
- Cost Efficiency: With fewer resources consumed for database operations, organizations can save on infrastructure costs, particularly for cloud-hosted systems.
For high-traffic APIs, preloading popular endpoints with anticipated data using cron jobs or scheduled tasks helps minimize cache misses. However, avoid caching highly dynamic data like real-time metrics, as frequent invalidations can decrease efficiency and add unnecessary complexity.
Common Challenges in Implementing Caching
- Cache Invalidation: Ensuring that cached data reflects the latest database state is critical. Without proper invalidation, users may receive outdated data.
- Stale Data Issues: Serving outdated information can lead to incorrect application behavior, especially in dynamic systems.
- Consistency Concerns: Synchronizing data between cache layers and the underlying database can be complex, particularly in distributed systems.
- Management Complexity: Configuring, monitoring, and maintaining cache systems requires careful planning and expertise..
Strategies for Effective Caching
Caching is a vital tool for improving API performance, but its success depends on choosing the right strategies. Different approaches, eviction policies, and granularity affect how effectively your application handles data. Below is a detailed explanation, incorporating best practices and the role of key features like headers and caching controls.
Choosing the Right Caching Strategy
- Write-Through Caching
- When data is updated or created, it is written to both the cache and the database simultaneously. This ensures that the cache and database remain consistent.
- Pros: Ensures data consistency between cache and database.
- Cons: Adds write latency because each create or update operation involves both the cache and the database.
- Use Case: Ideal for systems where consistency is critical, such as financial applications.
- Tip: Use a cache-control header with appropriate directives to ensure cached responses align with the expected freshness of data.
- Write-Behind Caching
- Data is written to the cache first and then asynchronously updated in the database. This reduces the latency for write operations but introduces a risk of data loss if the cache fails before syncing.
- Pros: Faster writes and reduced database load.
- Cons: Risk of data inconsistency or loss during failures.
- Use Case: Effective for scenarios where high write performance is essential, like logging systems.
- Best Practice: Ensure your application monitors cache write operations and employs mechanisms to retry failed updates to the database.
- Cache-Aside (Lazy Loading)
- Data is retrieved from the database and written to the cache only when it is absent (cache miss). Subsequent requests fetch the data directly from the cache.
- Pros: Efficient for read-heavy workloads, as only necessary data is cached.
- Cons: Potential latency during the first request (cache miss).
- Use Case: Common in systems where data is accessed infrequently but benefits from being cached when retrieved.
- Tip: Use the
cache-control
header to specify TTLs for cached responses, balancing freshness and resource usage.
Cache Eviction Policies
- Least Recently Used (LRU)
- Removes the least recently accessed data to make room for new entries when the cache reaches capacity.
- Pros: Retains frequently accessed data, optimizing bandwidth by reducing unnecessary database queries.
- Cons: Can be less effective for workloads with unpredictable access patterns.
- Use Case: General-purpose caching where usage patterns favor frequently accessed data.
- First In, First Out (FIFO)
- Evicts the oldest data first, regardless of access frequency.
- Pros: Simple and predictable eviction policy.
- Cons: May remove still-relevant data, increasing the chance of cache misses.
- Use Case: Useful when access patterns are tied to temporal order, such as log-based systems.
- Time-To-Live (TTL) Settings
- Automatically removes cached data after a specified duration, ensuring data freshness.
- Pros: Prevents stale data from persisting in the cache.
- Cons: Requires careful configuration to avoid excessive invalidation or outdated cached responses.
- Use Case: Systems where data changes frequently but benefits from short-term caching, such as stock prices or news feeds.
Granular Caching
- Fine-Grained Control
- Instead of caching entire API responses, focus on specific data fields or fragments.
- Pros: Reduces memory usage and ensures only the most valuable data is cached.
- Cons: Requires detailed analysis of access patterns and a more sophisticated cache management strategy.
- Use Case: APIs with large payloads, such as e-commerce product catalogs, where only a subset of data (e.g., product names and prices) is frequently accessed.
- Best Practice: Use conditional headers like If-None-Match or
Last-Modified
with cache-control to determine whether cached fragments are still valid.
Implementing Headers for Caching Control
When configuring caching strategies, headers play a critical role in defining cache behavior:
cache-control
Header: Allows fine-grained control over how cached responses are stored and invalidated. Common directives include:max-age:
Specifies the maximum time a cached response is valid.no-cache:
Ensures the cache must revalidate with the origin server before serving a response.must-revalidate:
Requires the cache to revalidate expired responses before use.
- Conditional Headers:
If-Modified-Since:
Reduces bandwidth by validating whether a cached response is still current based on the resource's last modification date.If-None-Match:
Compares the ETag of a cached response with the origin server's current version, avoiding unnecessary data transfer.
Integration with CRUD Operations
Caching should integrate seamlessly with core API operations (create, read, update, delete):
- Create (
PUT
orPOST
): Ensure new data is immediately cached using a write-through or write-behind approach. - Read (
GET
): Optimize performance by using cached responses and applying appropriate headers to control cache validity. - Update (
PUT
orPATCH
): Invalidate or refresh cache entries to prevent serving outdated data. Use event-driven invalidation to automate this process. - Delete (
DELETE
): Remove corresponding cache entries immediately to maintain consistency across the system.
Best Practices for API Caching
Identify What to Cache
Not all data or operations are equally cacheable. Focus on:
- Frequently Accessed Data: Cache data that is repeatedly retrieved through
GET
requests. Examples include product catalogs, user profiles, or static assets. - High Computation Costs: Endpoints involving heavy computations, such as analytics summaries, are excellent candidates for caching to minimize server load.
- Cache Keys: Use well-structured and unique cache keys for efficient data retrieval. For instance, a key like
user_123_profile
is better than a genericuser_profile
.
Example: In an e-commerce application using Amazon's AWS services, caching frequently accessed product data reduces the number of database queries, improving the next user's response time significantly.
Set Appropriate TTLs
The Time-To-Live (TTL) setting determines how long cached data remains valid:
- Dynamic Data: Set short TTLs to ensure freshness, especially for rapidly changing data like stock prices or session tokens.
- Static Data: Use longer TTLs for data that seldom changes, such as configuration settings or product descriptions.
- Balancing Act: Short TTLs ensure data freshness but may increase server load due to frequent revalidation. Long TTLs reduce server load but may serve outdated responses.
Tip: Pair TTL settings with a cache-control
header for granular control over cache expiration and revalidation in REST APIs.
Monitor and Analyze Cache Performance
Monitoring is essential for optimizing cache configurations and maintaining efficiency. Key metrics include:
- Cache Hit Rate: The percentage of requests served from the cache. A high hit rate indicates effective caching.
- Cache Misses: Analyze missed requests to identify unoptimized cache keys or insufficient TTL settings.
- Eviction Rates: Monitor how often cached entries are evicted due to capacity constraints.
Tools like Grafana or built-in monitoring features in Redis and Memcached can provide detailed insights into cache performance. By analyzing these metrics, you can adjust caching policies to improve resource utilization.
Security Considerations
Caching sensitive or personal data requires special attention to avoid data leaks:
- Encryption: Encrypt sensitive cached data, such as authentication tokens, to prevent unauthorized access.
- Access Control: Restrict cache access to trusted environments using authentication mechanisms.
- Client-Side Caching: Avoid caching sensitive data on the client side, as browsers or mobile apps may lack adequate security measures.
Example: When building a REST API for an authentication service, cache session tokens securely using Redis with encryption and strict access control.
Avoid Over-Caching
Over-caching can lead to resource wastage and stale data issues:
- Selective Caching: Cache only frequently accessed and computationally expensive data. Avoid caching ephemeral or low-priority information.
- Granular Caching: Instead of caching entire API responses, cache specific fields or fragments that are commonly requested.
Tip: Use cacheable directives in the cache-control
header to guide clients and intermediaries on what is appropriate to cache.
Tools and Technologies for Caching
- Redis
- High-Performance: An in-memory caching solution optimized for speed and advanced data structures.
- Use Case: Ideal for real-time applications like chat systems, session storage, or leaderboard tracking.
- Example: Cache frequently accessed REST API responses, such as
GET /user/123
for user profiles, using structured cache keys to minimize lookup time.
- Memcached
- Lightweight and Simple: A streamlined in-memory cache for storing small, transient chunks of data.
- Use Case: Best for short-term caching needs, such as temporary API response data or rate-limiting counters.
- Example: Cache authentication rate-limit counters for
POST /login
requests to reduce database queries.
- CDNs for Edge Caching
- Global Optimization: Content Delivery Networks (CDNs) like Amazon CloudFront cache data at edge locations close to users, significantly reducing latency.
- Use Case: Cache static assets and REST API responses that are client-facing and require high availability globally.
- Example: Cache product images and metadata using a CDN to offload traffic from the server and improve the next user's experience.
- API Integration
- Simplified Caching: API management tools like DreamFactory offer built-in caching capabilities to streamline integration.
- Use Case: Integrate cache policies directly into your REST API's infrastructure to ensure consistency and performance without manual configuration.
- Example: Use DreamFactory's caching to prefetch and cache database queries for a
GET /products
endpoint, reducing backend server load.
Conclusion
Caching is a key player in making APIs run smoothly, helping them scale up, respond faster, and save on costs. By getting to know the challenges and embracing best practices, developers can really tap into the awesome benefits of caching.
Check out tools like DreamFactory to easily weave caching into your API setup, all in your own environment!
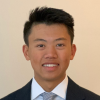
As a seasoned content moderator with a keen eye for detail and a passion for upholding the highest standards of quality and integrity in all of their work, Spencer Nguyen brings a professional yet empathetic approach to every task.